Sequelize - How to create/update record with N:M associations (create/update them too)How can I create a two dimensional array in JavaScript?How can I do an UPDATE statement with JOIN in SQL?How do I UPDATE from a SELECT in SQL Server?How can I update NodeJS and NPM to the next versions?How to update a record using sequelize for node?How do I update Node.js?How do I update each dependency in package.json to the latest version?How can I update npm on Windows?sequelize create or update with associationsequelize include, join two associated tables
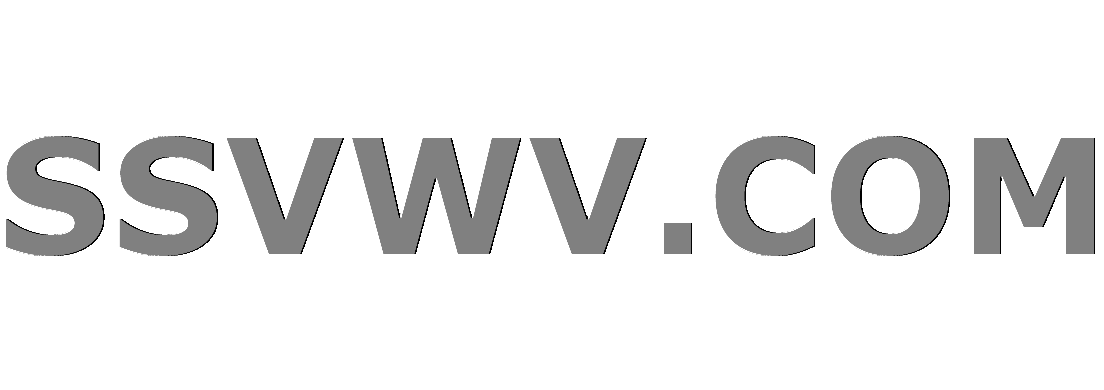
Multi tool use
How is the Apple Watch ECG disabled in certain countries?
How do we know neutrons have no charge?
Can RPi4 run simultaneously on dual band (WiFi 2.4GHz / 5GHz)?
What does it mean by "my days-of-the-week underwear only go to Thursday" in this context?
Can you cure a Gorgon's Petrifying Breath before it finishes turning a target to stone?
How to study endgames?
Can I exile my opponent's Progenitus/True-Name Nemesis with Teferi, Hero of Dominaria's emblem?
Problem updating custom Account custom fields with trigger
How important is knowledge of trig identities for use in Calculus
Can an energy drink or chocolate before an exam be useful ? What sort of other edible goods be helpful?
What is the meaning of colored vials next to some passive skills
A famous scholar sent me an unpublished draft of hers. Then she died. I think her work should be published. What should I do?
Top off gas with old oil, is that bad?
Assembly of PCBs containing a mix of SMT and thru-hole parts?
Differences between past simple + future simple /future in the past
A cotton-y connection
Garage door sticks on a bolt
Contour integration with infinite poles
Is there a relationship between prime numbers and music?
How to export all graphics from a notebook?
As a team leader is it appropriate to bring in fundraiser candy?
Worlds with different mathematics and logic
I transpose the source code, you transpose the input!
Why most footers have a background color has a divider of section?
Sequelize - How to create/update record with N:M associations (create/update them too)
How can I create a two dimensional array in JavaScript?How can I do an UPDATE statement with JOIN in SQL?How do I UPDATE from a SELECT in SQL Server?How can I update NodeJS and NPM to the next versions?How to update a record using sequelize for node?How do I update Node.js?How do I update each dependency in package.json to the latest version?How can I update npm on Windows?sequelize create or update with associationsequelize include, join two associated tables
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty margin-bottom:0;
I have Sequelize database model like so (simplified):
// Schedule model
const Schedule = sequelize.define('Schedule',
capacity:
type: DataTypes.INTEGER,
allowNull: false
,
,
hooks:
);
// Age category model
const AgeCategory = sequelize.define('AgeCategory',
key:
type: DataTypes.STRING,
unique: true,
allowNull: false
,
value:
type: DataTypes.STRING,
allowNull: false
);
// Many-to-many association
Schedule.associate = models =>
// Schedule have many AgeCategories
Schedule.belongsToMany(AgeCategory,
through: 'schedule_ageCategory',
foreignKey: 'schedule_id'
);
;
AgeCategory.associate = models =>
// Many AgeCategories are in many Schedules
AgeCategory.belongsToMany(Schedule,
through: 'schedule_ageCategory',
foreignKey: 'ageCategory_id',
onDelete: 'restrict',
onUpdate: 'restrict'
);
;
Create: When I creating new record I am doing this:
const data =
capacity: 10,
ageCategories: ["EXTRAOLD","YOUNG"] // 'key' from AgeCategory
;
const transaction = await sequelize.transaction();
let schedule = await Schedule.create(data,
isNewRecord: true,
transaction
);
// Set AgeCategories
await schedule.setAgeCategories(await AgeCategory.findAll(
where:
key: in: data.ageCategories
,
transaction
), transaction);
await transaction.commit();
Is there any better way to do it?
I am lost when I need to update Schedule (for example I need to set different age categories). How am I supposted to update n:m association? Lets say I have input data formatted like this:
const data =
capacity: 1,
ageCategories: ["OLD","YOUNG","DEAD"] // 'key' from AgeCategory
;
In this particular example it means I will delete one association, and make two new one.
javascript sql node.js sequelize.js
add a comment
|
I have Sequelize database model like so (simplified):
// Schedule model
const Schedule = sequelize.define('Schedule',
capacity:
type: DataTypes.INTEGER,
allowNull: false
,
,
hooks:
);
// Age category model
const AgeCategory = sequelize.define('AgeCategory',
key:
type: DataTypes.STRING,
unique: true,
allowNull: false
,
value:
type: DataTypes.STRING,
allowNull: false
);
// Many-to-many association
Schedule.associate = models =>
// Schedule have many AgeCategories
Schedule.belongsToMany(AgeCategory,
through: 'schedule_ageCategory',
foreignKey: 'schedule_id'
);
;
AgeCategory.associate = models =>
// Many AgeCategories are in many Schedules
AgeCategory.belongsToMany(Schedule,
through: 'schedule_ageCategory',
foreignKey: 'ageCategory_id',
onDelete: 'restrict',
onUpdate: 'restrict'
);
;
Create: When I creating new record I am doing this:
const data =
capacity: 10,
ageCategories: ["EXTRAOLD","YOUNG"] // 'key' from AgeCategory
;
const transaction = await sequelize.transaction();
let schedule = await Schedule.create(data,
isNewRecord: true,
transaction
);
// Set AgeCategories
await schedule.setAgeCategories(await AgeCategory.findAll(
where:
key: in: data.ageCategories
,
transaction
), transaction);
await transaction.commit();
Is there any better way to do it?
I am lost when I need to update Schedule (for example I need to set different age categories). How am I supposted to update n:m association? Lets say I have input data formatted like this:
const data =
capacity: 1,
ageCategories: ["OLD","YOUNG","DEAD"] // 'key' from AgeCategory
;
In this particular example it means I will delete one association, and make two new one.
javascript sql node.js sequelize.js
Hi. The sequelize has a manual explaining the best way to do this. You can see at: sequelize.readthedocs.io/en/v3/api/associations/belongs-to-many
– rpereira15
Mar 28 at 21:41
I know but I don't understand it very well
– David Bubeník
Mar 29 at 9:51
add a comment
|
I have Sequelize database model like so (simplified):
// Schedule model
const Schedule = sequelize.define('Schedule',
capacity:
type: DataTypes.INTEGER,
allowNull: false
,
,
hooks:
);
// Age category model
const AgeCategory = sequelize.define('AgeCategory',
key:
type: DataTypes.STRING,
unique: true,
allowNull: false
,
value:
type: DataTypes.STRING,
allowNull: false
);
// Many-to-many association
Schedule.associate = models =>
// Schedule have many AgeCategories
Schedule.belongsToMany(AgeCategory,
through: 'schedule_ageCategory',
foreignKey: 'schedule_id'
);
;
AgeCategory.associate = models =>
// Many AgeCategories are in many Schedules
AgeCategory.belongsToMany(Schedule,
through: 'schedule_ageCategory',
foreignKey: 'ageCategory_id',
onDelete: 'restrict',
onUpdate: 'restrict'
);
;
Create: When I creating new record I am doing this:
const data =
capacity: 10,
ageCategories: ["EXTRAOLD","YOUNG"] // 'key' from AgeCategory
;
const transaction = await sequelize.transaction();
let schedule = await Schedule.create(data,
isNewRecord: true,
transaction
);
// Set AgeCategories
await schedule.setAgeCategories(await AgeCategory.findAll(
where:
key: in: data.ageCategories
,
transaction
), transaction);
await transaction.commit();
Is there any better way to do it?
I am lost when I need to update Schedule (for example I need to set different age categories). How am I supposted to update n:m association? Lets say I have input data formatted like this:
const data =
capacity: 1,
ageCategories: ["OLD","YOUNG","DEAD"] // 'key' from AgeCategory
;
In this particular example it means I will delete one association, and make two new one.
javascript sql node.js sequelize.js
I have Sequelize database model like so (simplified):
// Schedule model
const Schedule = sequelize.define('Schedule',
capacity:
type: DataTypes.INTEGER,
allowNull: false
,
,
hooks:
);
// Age category model
const AgeCategory = sequelize.define('AgeCategory',
key:
type: DataTypes.STRING,
unique: true,
allowNull: false
,
value:
type: DataTypes.STRING,
allowNull: false
);
// Many-to-many association
Schedule.associate = models =>
// Schedule have many AgeCategories
Schedule.belongsToMany(AgeCategory,
through: 'schedule_ageCategory',
foreignKey: 'schedule_id'
);
;
AgeCategory.associate = models =>
// Many AgeCategories are in many Schedules
AgeCategory.belongsToMany(Schedule,
through: 'schedule_ageCategory',
foreignKey: 'ageCategory_id',
onDelete: 'restrict',
onUpdate: 'restrict'
);
;
Create: When I creating new record I am doing this:
const data =
capacity: 10,
ageCategories: ["EXTRAOLD","YOUNG"] // 'key' from AgeCategory
;
const transaction = await sequelize.transaction();
let schedule = await Schedule.create(data,
isNewRecord: true,
transaction
);
// Set AgeCategories
await schedule.setAgeCategories(await AgeCategory.findAll(
where:
key: in: data.ageCategories
,
transaction
), transaction);
await transaction.commit();
Is there any better way to do it?
I am lost when I need to update Schedule (for example I need to set different age categories). How am I supposted to update n:m association? Lets say I have input data formatted like this:
const data =
capacity: 1,
ageCategories: ["OLD","YOUNG","DEAD"] // 'key' from AgeCategory
;
In this particular example it means I will delete one association, and make two new one.
javascript sql node.js sequelize.js
javascript sql node.js sequelize.js
asked Mar 28 at 19:52


David BubeníkDavid Bubeník
5310 bronze badges
5310 bronze badges
Hi. The sequelize has a manual explaining the best way to do this. You can see at: sequelize.readthedocs.io/en/v3/api/associations/belongs-to-many
– rpereira15
Mar 28 at 21:41
I know but I don't understand it very well
– David Bubeník
Mar 29 at 9:51
add a comment
|
Hi. The sequelize has a manual explaining the best way to do this. You can see at: sequelize.readthedocs.io/en/v3/api/associations/belongs-to-many
– rpereira15
Mar 28 at 21:41
I know but I don't understand it very well
– David Bubeník
Mar 29 at 9:51
Hi. The sequelize has a manual explaining the best way to do this. You can see at: sequelize.readthedocs.io/en/v3/api/associations/belongs-to-many
– rpereira15
Mar 28 at 21:41
Hi. The sequelize has a manual explaining the best way to do this. You can see at: sequelize.readthedocs.io/en/v3/api/associations/belongs-to-many
– rpereira15
Mar 28 at 21:41
I know but I don't understand it very well
– David Bubeník
Mar 29 at 9:51
I know but I don't understand it very well
– David Bubeník
Mar 29 at 9:51
add a comment
|
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/4.0/"u003ecc by-sa 4.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f55405847%2fsequelize-how-to-create-update-record-with-nm-associations-create-update-the%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f55405847%2fsequelize-how-to-create-update-record-with-nm-associations-create-update-the%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Rqvet,gqwt5JbEj
Hi. The sequelize has a manual explaining the best way to do this. You can see at: sequelize.readthedocs.io/en/v3/api/associations/belongs-to-many
– rpereira15
Mar 28 at 21:41
I know but I don't understand it very well
– David Bubeník
Mar 29 at 9:51