Changing the face and moods in python turtleCalling an external command in PythonWhat are metaclasses in Python?Finding the index of an item given a list containing it in PythonWhat is the difference between Python's list methods append and extend?How can I safely create a nested directory?Does Python have a ternary conditional operator?How to get the current time in PythonHow can I make a time delay in Python?Does Python have a string 'contains' substring method?Why is “1000000000000000 in range(1000000000000001)” so fast in Python 3?
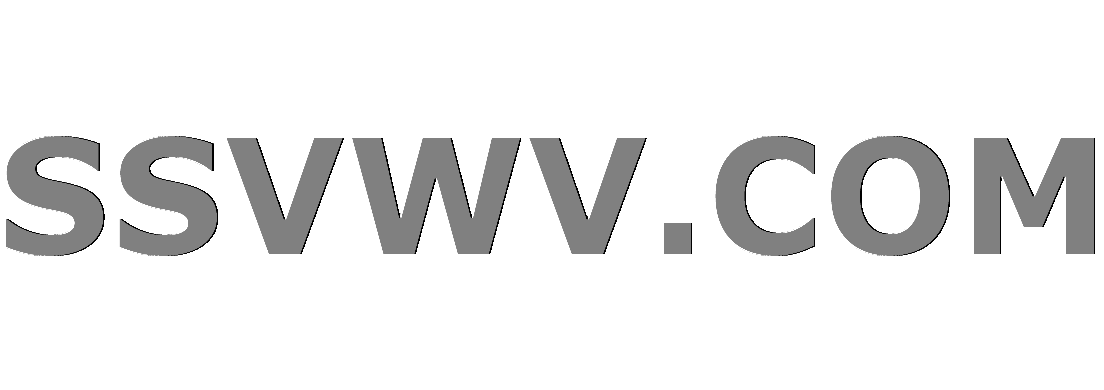
Multi tool use
Do empty drive bays need to be filled?
What are the unintended or dangerous consequences of allowing spells that target and damage creatures to also target and damage objects?
Who is "He that flies" in Lord of the Rings?
Why is the length of the Kelvin unit of temperature equal to that of the Celsius unit?
Why is long-term living in Almost-Earth causing severe health problems?
So a part of my house disappeared... But not because of a chunk resetting
How do we say "within a kilometer radius spherically"?
bash vs. zsh: What are the practical differences?
I've been given a project I can't complete, what should I do?
Should I refuse to be named as co-author of a low quality paper?
How (un)safe is it to ride barefoot?
What would be the way to say "just saying" in German? (Not the literal translation)
Assigning function to function pointer, const argument correctness?
How to write a convincing religious myth?
Command of files and size
Is there a DSLR/mirorless camera with minimal options like a classic, simple SLR?
Why do radiation hardened IC packages often have long leads?
What do Birth, Age, and Death mean in the first noble truth?
What STL algorithm can determine if exactly one item in a container satisfies a predicate?
Converting from CMYK to RGB (to work with it), then back to CMYK
What do you call the action of "describing events as they happen" like sports anchors do?
Trying to get (more) accurate readings from thermistor (electronics, math, and code inside)
Breaking changes to eieio in Emacs 27?
Grep Match and extract
Changing the face and moods in python turtle
Calling an external command in PythonWhat are metaclasses in Python?Finding the index of an item given a list containing it in PythonWhat is the difference between Python's list methods append and extend?How can I safely create a nested directory?Does Python have a ternary conditional operator?How to get the current time in PythonHow can I make a time delay in Python?Does Python have a string 'contains' substring method?Why is “1000000000000000 in range(1000000000000001)” so fast in Python 3?
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty height:90px;width:728px;box-sizing:border-box;
The user supposed to be prompted to change the face with the following menu:
Change My Face
1) Make me frown
2) Make me angry
3) Make my eyes blue
0) Quit
If the user selects 1, the smiley face is redrawn with the frown turned to a smile and the menu will change to:
Change My Face
1) Make me smile
2) Make me angry
3) Make my eyes blue
0) Quit
If the user selects 2, the smiley face is redrawn and filled in red with a frown and the menu will change to:
Change My Face
1) Make me smile
2) Make me happy
3) Make my eyes blue
0) Quit
We were given starter code to work on this project (with everywhere we are to add a single line/partial line of code). We also need to and can add methods and data as necessary to this existing code. I am having a hard time understanding class and objects, and this is proving to be a more difficult assignment for me than I originally thought it would be. Here is the starter code we were given (we are defining class in the same file as our main function for convenience):
import turtle
class Face:
def __init__(self):
self.__smile = True
self.__happy = True
self.__dark_eyes = True
def draw_face(self):
turtle.clear()
self.__draw_head()
self.__draw_eyes()
self.__draw_mouth()
def is_smile(self):
___<Fill-In>___
def is_happy(self):
___<Fill-In>___
def is_dark_eyes(self):
___<Fill-In>___
def change_mouth(self):
___<Fill-In>___
self.draw_face()
def change_emotion(self):
___<Fill-In>___
self.draw_face()
def change_eyes(self):
___<Fill-In>___
self.draw_face()
def main():
face = ___<Fill-In>___
face.___<Fill-In>___
done = False
while not done:
print("Change My Face")
mouth = "frown" ____<Fill-In>___ "smile"
emotion = "angry" ____<Fill-In>___ "happy"
eyes = "blue" ____<Fill-In>___ "black"
print("1) Make me", mouth)
print("2) Make me", emotion)
print("3) Make my eyes", eyes)
print("0) Quit")
menu = eval(input("Enter a selection: "))
if menu == 1:
___<Fill-In>___
elif menu == 2:
___<Fill-In>___
elif menu == 3:
___<Fill-In>___
else:
break
print("Thanks for Playing")
turtle.hideturtle()
turtle.done()
main()
I am guessing that should use if else for the fill ins, but other than that I am not sure what any other fill in would be.
while not done:
print("Change My Face")
mouth = "frown" ____<Fill-In>___ "smile"
emotion = "angry" ____<Fill-In>___ "happy"
eyes = "blue" ____<Fill-In>___ "black"
print("1) Make me", mouth)
print("2) Make me", emotion)
print("3) Make my eyes", eyes)
print("0) Quit")
Thank you for your help!
python python-3.x
add a comment |
The user supposed to be prompted to change the face with the following menu:
Change My Face
1) Make me frown
2) Make me angry
3) Make my eyes blue
0) Quit
If the user selects 1, the smiley face is redrawn with the frown turned to a smile and the menu will change to:
Change My Face
1) Make me smile
2) Make me angry
3) Make my eyes blue
0) Quit
If the user selects 2, the smiley face is redrawn and filled in red with a frown and the menu will change to:
Change My Face
1) Make me smile
2) Make me happy
3) Make my eyes blue
0) Quit
We were given starter code to work on this project (with everywhere we are to add a single line/partial line of code). We also need to and can add methods and data as necessary to this existing code. I am having a hard time understanding class and objects, and this is proving to be a more difficult assignment for me than I originally thought it would be. Here is the starter code we were given (we are defining class in the same file as our main function for convenience):
import turtle
class Face:
def __init__(self):
self.__smile = True
self.__happy = True
self.__dark_eyes = True
def draw_face(self):
turtle.clear()
self.__draw_head()
self.__draw_eyes()
self.__draw_mouth()
def is_smile(self):
___<Fill-In>___
def is_happy(self):
___<Fill-In>___
def is_dark_eyes(self):
___<Fill-In>___
def change_mouth(self):
___<Fill-In>___
self.draw_face()
def change_emotion(self):
___<Fill-In>___
self.draw_face()
def change_eyes(self):
___<Fill-In>___
self.draw_face()
def main():
face = ___<Fill-In>___
face.___<Fill-In>___
done = False
while not done:
print("Change My Face")
mouth = "frown" ____<Fill-In>___ "smile"
emotion = "angry" ____<Fill-In>___ "happy"
eyes = "blue" ____<Fill-In>___ "black"
print("1) Make me", mouth)
print("2) Make me", emotion)
print("3) Make my eyes", eyes)
print("0) Quit")
menu = eval(input("Enter a selection: "))
if menu == 1:
___<Fill-In>___
elif menu == 2:
___<Fill-In>___
elif menu == 3:
___<Fill-In>___
else:
break
print("Thanks for Playing")
turtle.hideturtle()
turtle.done()
main()
I am guessing that should use if else for the fill ins, but other than that I am not sure what any other fill in would be.
while not done:
print("Change My Face")
mouth = "frown" ____<Fill-In>___ "smile"
emotion = "angry" ____<Fill-In>___ "happy"
eyes = "blue" ____<Fill-In>___ "black"
print("1) Make me", mouth)
print("2) Make me", emotion)
print("3) Make my eyes", eyes)
print("0) Quit")
Thank you for your help!
python python-3.x
It's off topic but I'd be dubious of any Python 3 instructor or book that gives you starter code likemenu = eval(input("Enter a selection: "))
. There's no excuse for aneval()
, vs. anint()
, here. Back on topic, you really need to provide the code you've added so far, and tell us why it's not working, otherwise you're just posting your homework assignment for other folks to work on..
– cdlane
Mar 25 at 1:35
Hello! I am looking for ideas as to what he is looking for in the fill in area. That is the area where I am stuck. I think I could get it after that. Would that be where you draw your face and such?
– madison2000
Mar 25 at 3:10
add a comment |
The user supposed to be prompted to change the face with the following menu:
Change My Face
1) Make me frown
2) Make me angry
3) Make my eyes blue
0) Quit
If the user selects 1, the smiley face is redrawn with the frown turned to a smile and the menu will change to:
Change My Face
1) Make me smile
2) Make me angry
3) Make my eyes blue
0) Quit
If the user selects 2, the smiley face is redrawn and filled in red with a frown and the menu will change to:
Change My Face
1) Make me smile
2) Make me happy
3) Make my eyes blue
0) Quit
We were given starter code to work on this project (with everywhere we are to add a single line/partial line of code). We also need to and can add methods and data as necessary to this existing code. I am having a hard time understanding class and objects, and this is proving to be a more difficult assignment for me than I originally thought it would be. Here is the starter code we were given (we are defining class in the same file as our main function for convenience):
import turtle
class Face:
def __init__(self):
self.__smile = True
self.__happy = True
self.__dark_eyes = True
def draw_face(self):
turtle.clear()
self.__draw_head()
self.__draw_eyes()
self.__draw_mouth()
def is_smile(self):
___<Fill-In>___
def is_happy(self):
___<Fill-In>___
def is_dark_eyes(self):
___<Fill-In>___
def change_mouth(self):
___<Fill-In>___
self.draw_face()
def change_emotion(self):
___<Fill-In>___
self.draw_face()
def change_eyes(self):
___<Fill-In>___
self.draw_face()
def main():
face = ___<Fill-In>___
face.___<Fill-In>___
done = False
while not done:
print("Change My Face")
mouth = "frown" ____<Fill-In>___ "smile"
emotion = "angry" ____<Fill-In>___ "happy"
eyes = "blue" ____<Fill-In>___ "black"
print("1) Make me", mouth)
print("2) Make me", emotion)
print("3) Make my eyes", eyes)
print("0) Quit")
menu = eval(input("Enter a selection: "))
if menu == 1:
___<Fill-In>___
elif menu == 2:
___<Fill-In>___
elif menu == 3:
___<Fill-In>___
else:
break
print("Thanks for Playing")
turtle.hideturtle()
turtle.done()
main()
I am guessing that should use if else for the fill ins, but other than that I am not sure what any other fill in would be.
while not done:
print("Change My Face")
mouth = "frown" ____<Fill-In>___ "smile"
emotion = "angry" ____<Fill-In>___ "happy"
eyes = "blue" ____<Fill-In>___ "black"
print("1) Make me", mouth)
print("2) Make me", emotion)
print("3) Make my eyes", eyes)
print("0) Quit")
Thank you for your help!
python python-3.x
The user supposed to be prompted to change the face with the following menu:
Change My Face
1) Make me frown
2) Make me angry
3) Make my eyes blue
0) Quit
If the user selects 1, the smiley face is redrawn with the frown turned to a smile and the menu will change to:
Change My Face
1) Make me smile
2) Make me angry
3) Make my eyes blue
0) Quit
If the user selects 2, the smiley face is redrawn and filled in red with a frown and the menu will change to:
Change My Face
1) Make me smile
2) Make me happy
3) Make my eyes blue
0) Quit
We were given starter code to work on this project (with everywhere we are to add a single line/partial line of code). We also need to and can add methods and data as necessary to this existing code. I am having a hard time understanding class and objects, and this is proving to be a more difficult assignment for me than I originally thought it would be. Here is the starter code we were given (we are defining class in the same file as our main function for convenience):
import turtle
class Face:
def __init__(self):
self.__smile = True
self.__happy = True
self.__dark_eyes = True
def draw_face(self):
turtle.clear()
self.__draw_head()
self.__draw_eyes()
self.__draw_mouth()
def is_smile(self):
___<Fill-In>___
def is_happy(self):
___<Fill-In>___
def is_dark_eyes(self):
___<Fill-In>___
def change_mouth(self):
___<Fill-In>___
self.draw_face()
def change_emotion(self):
___<Fill-In>___
self.draw_face()
def change_eyes(self):
___<Fill-In>___
self.draw_face()
def main():
face = ___<Fill-In>___
face.___<Fill-In>___
done = False
while not done:
print("Change My Face")
mouth = "frown" ____<Fill-In>___ "smile"
emotion = "angry" ____<Fill-In>___ "happy"
eyes = "blue" ____<Fill-In>___ "black"
print("1) Make me", mouth)
print("2) Make me", emotion)
print("3) Make my eyes", eyes)
print("0) Quit")
menu = eval(input("Enter a selection: "))
if menu == 1:
___<Fill-In>___
elif menu == 2:
___<Fill-In>___
elif menu == 3:
___<Fill-In>___
else:
break
print("Thanks for Playing")
turtle.hideturtle()
turtle.done()
main()
I am guessing that should use if else for the fill ins, but other than that I am not sure what any other fill in would be.
while not done:
print("Change My Face")
mouth = "frown" ____<Fill-In>___ "smile"
emotion = "angry" ____<Fill-In>___ "happy"
eyes = "blue" ____<Fill-In>___ "black"
print("1) Make me", mouth)
print("2) Make me", emotion)
print("3) Make my eyes", eyes)
print("0) Quit")
Thank you for your help!
python python-3.x
python python-3.x
edited Mar 25 at 3:17


eyllanesc
95.6k123769
95.6k123769
asked Mar 24 at 21:49
madison2000madison2000
63
63
It's off topic but I'd be dubious of any Python 3 instructor or book that gives you starter code likemenu = eval(input("Enter a selection: "))
. There's no excuse for aneval()
, vs. anint()
, here. Back on topic, you really need to provide the code you've added so far, and tell us why it's not working, otherwise you're just posting your homework assignment for other folks to work on..
– cdlane
Mar 25 at 1:35
Hello! I am looking for ideas as to what he is looking for in the fill in area. That is the area where I am stuck. I think I could get it after that. Would that be where you draw your face and such?
– madison2000
Mar 25 at 3:10
add a comment |
It's off topic but I'd be dubious of any Python 3 instructor or book that gives you starter code likemenu = eval(input("Enter a selection: "))
. There's no excuse for aneval()
, vs. anint()
, here. Back on topic, you really need to provide the code you've added so far, and tell us why it's not working, otherwise you're just posting your homework assignment for other folks to work on..
– cdlane
Mar 25 at 1:35
Hello! I am looking for ideas as to what he is looking for in the fill in area. That is the area where I am stuck. I think I could get it after that. Would that be where you draw your face and such?
– madison2000
Mar 25 at 3:10
It's off topic but I'd be dubious of any Python 3 instructor or book that gives you starter code like
menu = eval(input("Enter a selection: "))
. There's no excuse for an eval()
, vs. an int()
, here. Back on topic, you really need to provide the code you've added so far, and tell us why it's not working, otherwise you're just posting your homework assignment for other folks to work on..– cdlane
Mar 25 at 1:35
It's off topic but I'd be dubious of any Python 3 instructor or book that gives you starter code like
menu = eval(input("Enter a selection: "))
. There's no excuse for an eval()
, vs. an int()
, here. Back on topic, you really need to provide the code you've added so far, and tell us why it's not working, otherwise you're just posting your homework assignment for other folks to work on..– cdlane
Mar 25 at 1:35
Hello! I am looking for ideas as to what he is looking for in the fill in area. That is the area where I am stuck. I think I could get it after that. Would that be where you draw your face and such?
– madison2000
Mar 25 at 3:10
Hello! I am looking for ideas as to what he is looking for in the fill in area. That is the area where I am stuck. I think I could get it after that. Would that be where you draw your face and such?
– madison2000
Mar 25 at 3:10
add a comment |
1 Answer
1
active
oldest
votes
From top to bottom:
def is_smile(self):
___<Fill-In>___
In python methods that start with 'is' should return a boolean value after some evaluation.
I would guess your instructor wants you to return true if the face .smile attribute is true and false if its false.
def change_mouth(self):
___<Fill-In>___
self.draw_face()
This function seems like it wants you to change the mouth of the turtle. since it does not accept a value to change to, its safe to assume he wants you to change the value of smile from its current value to the logical alternative. since smile is a boolean, then change it from true to false or false to true.
def main():
face = ___<Fill-In>___
face.___<Fill-In>___
Ok were in the main application now, in order to have a face to play with you need to instantiate a new object of the Face class.
And then since the init method of the Face class does not do anything to draw the face on the screen it appears he wants you to call the method that draws the face.
while not done:
print("Change My Face")
mouth = "frown" ____<Fill-In>___ "smile"
emotion = "angry" ____<Fill-In>___ "happy"
eyes = "blue" ____<Fill-In>___ "black"
print("1) Make me", mouth)
print("2) Make me", emotion)
print("3) Make my eyes", eyes)
print("0) Quit")
an if else should work great for these. try calling those is_xxx functions you made above.
if menu == 1:
___<Fill-In>___
elif menu == 2:
___<Fill-In>___
elif menu == 3:
___<Fill-In>___
else:
break
You need to call the change methods you made above.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f55328908%2fchanging-the-face-and-moods-in-python-turtle%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
From top to bottom:
def is_smile(self):
___<Fill-In>___
In python methods that start with 'is' should return a boolean value after some evaluation.
I would guess your instructor wants you to return true if the face .smile attribute is true and false if its false.
def change_mouth(self):
___<Fill-In>___
self.draw_face()
This function seems like it wants you to change the mouth of the turtle. since it does not accept a value to change to, its safe to assume he wants you to change the value of smile from its current value to the logical alternative. since smile is a boolean, then change it from true to false or false to true.
def main():
face = ___<Fill-In>___
face.___<Fill-In>___
Ok were in the main application now, in order to have a face to play with you need to instantiate a new object of the Face class.
And then since the init method of the Face class does not do anything to draw the face on the screen it appears he wants you to call the method that draws the face.
while not done:
print("Change My Face")
mouth = "frown" ____<Fill-In>___ "smile"
emotion = "angry" ____<Fill-In>___ "happy"
eyes = "blue" ____<Fill-In>___ "black"
print("1) Make me", mouth)
print("2) Make me", emotion)
print("3) Make my eyes", eyes)
print("0) Quit")
an if else should work great for these. try calling those is_xxx functions you made above.
if menu == 1:
___<Fill-In>___
elif menu == 2:
___<Fill-In>___
elif menu == 3:
___<Fill-In>___
else:
break
You need to call the change methods you made above.
add a comment |
From top to bottom:
def is_smile(self):
___<Fill-In>___
In python methods that start with 'is' should return a boolean value after some evaluation.
I would guess your instructor wants you to return true if the face .smile attribute is true and false if its false.
def change_mouth(self):
___<Fill-In>___
self.draw_face()
This function seems like it wants you to change the mouth of the turtle. since it does not accept a value to change to, its safe to assume he wants you to change the value of smile from its current value to the logical alternative. since smile is a boolean, then change it from true to false or false to true.
def main():
face = ___<Fill-In>___
face.___<Fill-In>___
Ok were in the main application now, in order to have a face to play with you need to instantiate a new object of the Face class.
And then since the init method of the Face class does not do anything to draw the face on the screen it appears he wants you to call the method that draws the face.
while not done:
print("Change My Face")
mouth = "frown" ____<Fill-In>___ "smile"
emotion = "angry" ____<Fill-In>___ "happy"
eyes = "blue" ____<Fill-In>___ "black"
print("1) Make me", mouth)
print("2) Make me", emotion)
print("3) Make my eyes", eyes)
print("0) Quit")
an if else should work great for these. try calling those is_xxx functions you made above.
if menu == 1:
___<Fill-In>___
elif menu == 2:
___<Fill-In>___
elif menu == 3:
___<Fill-In>___
else:
break
You need to call the change methods you made above.
add a comment |
From top to bottom:
def is_smile(self):
___<Fill-In>___
In python methods that start with 'is' should return a boolean value after some evaluation.
I would guess your instructor wants you to return true if the face .smile attribute is true and false if its false.
def change_mouth(self):
___<Fill-In>___
self.draw_face()
This function seems like it wants you to change the mouth of the turtle. since it does not accept a value to change to, its safe to assume he wants you to change the value of smile from its current value to the logical alternative. since smile is a boolean, then change it from true to false or false to true.
def main():
face = ___<Fill-In>___
face.___<Fill-In>___
Ok were in the main application now, in order to have a face to play with you need to instantiate a new object of the Face class.
And then since the init method of the Face class does not do anything to draw the face on the screen it appears he wants you to call the method that draws the face.
while not done:
print("Change My Face")
mouth = "frown" ____<Fill-In>___ "smile"
emotion = "angry" ____<Fill-In>___ "happy"
eyes = "blue" ____<Fill-In>___ "black"
print("1) Make me", mouth)
print("2) Make me", emotion)
print("3) Make my eyes", eyes)
print("0) Quit")
an if else should work great for these. try calling those is_xxx functions you made above.
if menu == 1:
___<Fill-In>___
elif menu == 2:
___<Fill-In>___
elif menu == 3:
___<Fill-In>___
else:
break
You need to call the change methods you made above.
From top to bottom:
def is_smile(self):
___<Fill-In>___
In python methods that start with 'is' should return a boolean value after some evaluation.
I would guess your instructor wants you to return true if the face .smile attribute is true and false if its false.
def change_mouth(self):
___<Fill-In>___
self.draw_face()
This function seems like it wants you to change the mouth of the turtle. since it does not accept a value to change to, its safe to assume he wants you to change the value of smile from its current value to the logical alternative. since smile is a boolean, then change it from true to false or false to true.
def main():
face = ___<Fill-In>___
face.___<Fill-In>___
Ok were in the main application now, in order to have a face to play with you need to instantiate a new object of the Face class.
And then since the init method of the Face class does not do anything to draw the face on the screen it appears he wants you to call the method that draws the face.
while not done:
print("Change My Face")
mouth = "frown" ____<Fill-In>___ "smile"
emotion = "angry" ____<Fill-In>___ "happy"
eyes = "blue" ____<Fill-In>___ "black"
print("1) Make me", mouth)
print("2) Make me", emotion)
print("3) Make my eyes", eyes)
print("0) Quit")
an if else should work great for these. try calling those is_xxx functions you made above.
if menu == 1:
___<Fill-In>___
elif menu == 2:
___<Fill-In>___
elif menu == 3:
___<Fill-In>___
else:
break
You need to call the change methods you made above.
answered Mar 25 at 3:55
Chris ThompsonChris Thompson
31
31
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f55328908%2fchanging-the-face-and-moods-in-python-turtle%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
DKgP THP 3rLIk8iPqxuib1RPvajsMNDUEus7l8eUR nACUGpGc
It's off topic but I'd be dubious of any Python 3 instructor or book that gives you starter code like
menu = eval(input("Enter a selection: "))
. There's no excuse for aneval()
, vs. anint()
, here. Back on topic, you really need to provide the code you've added so far, and tell us why it's not working, otherwise you're just posting your homework assignment for other folks to work on..– cdlane
Mar 25 at 1:35
Hello! I am looking for ideas as to what he is looking for in the fill in area. That is the area where I am stuck. I think I could get it after that. Would that be where you draw your face and such?
– madison2000
Mar 25 at 3:10